Table of content
SHARE THIS ARTICLE
Is this blog hitting the mark?
Quick Overview: Node.js testing is like trying out a new recipe. It's about checking if the code for applications made with Node.js works well. It's just like making sure each ingredient in a recipe is doing its job.
There are different types of tests, just as there are different steps in a recipe. For instance, small tests (like checking each ingredient) are called unit tests. Then there are tests to see how different parts of the code work together, similar to mixing ingredients; these are called integration tests. Lastly, there are tests to see if the whole process works smoothly, like checking if the baked cake looks and tastes good—these are end-to-end tests.
To help with these tests, there are special tools, much like using a mixer or an oven in cooking. These tools are like Mocha, Jest, or Jasmine. They make it easier to write and run these tests.
Testing in Node.js is important because it helps find and fix mistakes early. Just as a chef tastes the dish while cooking to adjust the flavors, developers test their code to fix any issues before users face them. The goal is to ensure that the applications made with Node.js are strong, reliable, and work just as they're supposed to.
Table of Content
- Introduction to Node.js Testing
- Importance of Testing in Node.js Applications
- Types of Testing in Node.js
- Testing Tools and Frameworks
- Setting Up Testing Environment
- Writing Unit Tests in Node.js
- Conducting Integration Tests
- Implementing End-to-End Tests
- Best Practices for Node.js Testing
- Continuous Learning
- FAQs
Introduction to Node.js Testing
Node.js testing is like quality assurance for software. It involves running tests to ensure your code works without errors or issues. These tests check different parts of the code, making sure they function well both individually and together. Node.js testing tools help in this process, ensuring your application works as intended, just like how safety checks ensure a vehicle's reliability for the road. Overall, it's about ensuring that users can trust your software to function properly.
Importance of Testing in Node.js Applications
Testing holds immense significance in Node.js applications for several crucial reasons:
Error Detection: Testing helps in identifying errors, bugs, or unexpected behavior within the code. This early discovery allows developers to fix issues before they impact users or the application's performance.
Enhanced Code Quality: Thorough testing contributes to better code quality. It ensures that the code performs as intended, meets requirements, and adheres to best practices, resulting in more reliable and maintainable software.
Prevention of Issues: It aids in preventing issues that might arise in different environments or with various user interactions. By simulating these scenarios in testing, developers can proactively address potential problems.
Cost-Efficiency: Identifying and fixing issues in the early stages of development is more cost-effective than addressing them later in the production or post-release phase. Testing mitigates risks and reduces expenses associated with fixing larger issues.
User Satisfaction: A thoroughly tested application typically offers a better user experience. It ensures that the software functions correctly, enhancing user satisfaction and trust in the product.
Maintainability and Scalability: Well-tested code is easier to maintain and scale. As the codebase grows, testing ensures new features or modifications don't break existing functionality.
Supports Agile Development: In an agile development environment, testing allows for quicker iterations and the implementation of changes. It provides confidence to make alterations and improvements while maintaining the software's stability.
Compliance and Security: Testing helps in ensuring compliance with industry standards and security measures. It identifies vulnerabilities and potential security threats, enabling developers to fortify the application's security.
Also read: Importance of Performance Testing
Types of Testing in Node.js
Here are different types of testing in Node.js with corresponding examples:
Unit Testing:
Definition: Tests individual parts (units) of code in isolation, like functions or modules, to verify they work as expected.
Example: Testing a specific function that calculates the total cost in an e-commerce application to ensure it produces accurate results.
Integration Testing:
Definition: Checks how different parts of an application work together. It focuses on interactions between various modules or components.
Example: Testing the interaction between the database and the backend server to ensure data is retrieved and stored correctly.
End-to-End Testing:
Definition: Examines the entire flow of an application, simulating real user scenarios from start to finish. It tests the complete workflow.
Example: Testing the user registration, login, and purchase process in an online shopping app to ensure all steps work seamlessly.
Regression Testing:
Definition: Re-running tests to ensure that new code changes don't break existing functionalities that were previously working.
Example: After making updates to an application, running tests to confirm that previous features still work as expected.
Performance Testing:
Definition: Evaluates the speed, responsiveness, and stability of an application under different conditions, such as heavy loads or high traffic.
Example: Testing how an application handles simultaneous user requests to ensure it remains responsive without slowing down.
Testing Tools and Frameworks
1. Mocha
Type: Testing Framework
Description: A flexible and widely-used testing framework that supports various assertion libraries and different testing styles. Mocha is known for its extensive ecosystem of plugins and simple, expressive syntax.
Website: Mocha
2. Jest
Type: Testing Framework
Description: A JavaScript testing framework with built-in assertions, mocking, and a focus on simplicity. Jest is often used for testing Node.js applications as well as React applications.
Website: Jest
3. Chai
Type: Assertion Library
Description: Chai is an assertion library that pairs well with Mocha and other testing frameworks. It provides a wide range of assertion styles and helps make your tests more readable and expressive.
Website: Chai
4. Sinon
Type: Mocking and Stubbing Library
Description: Sinon is a powerful library for creating spies, mocks, and stubs. It's especially useful for testing functions with dependencies or external interactions.
Website: Sinon
5. Jasmine
Type: Testing Framework
Description: A behavior-driven development (BDD) testing framework that uses its own syntax for structuring tests. It's known for its readable and descriptive test suites.
Website: Jasmine
6. Istanbul (NYC)
Type: Test Coverage Tool
Description: Istanbul, often used through the nyc command-line tool, provides code coverage reports to identify areas of your codebase that are under-tested. It helps ensure comprehensive testing.
Website: Istanbul
Also read: Top 5 Automated Testing Tools for Web Apps (with Pros and Cons)
Setting Up Testing Environment
Install Node.js and NPM: Ensure that you have Node.js and NPM installed on your system. You can download and install them from the official Node.js website.
Create a Project Directory: Set up a dedicated directory for your Node.js project. Navigate to this directory in your terminal.
Initialize a Node.js project: Run “npm init -y” to create a “package.json” file in your project directory. This file contains project metadata and dependencies.
Install testing framework and tools: Choose and install the testing framework and tools you'll use. For instance, if you're using Mocha, Chai, and Sinon, install them as development dependencies:

Writing Unit Tests in Node.js
Create a Test File: Inside the "tests" directory, create a test file corresponding to the module or function you want to test. Follow a naming convention like “<module-name>. test.js”.
Write Unit Tests: In your test file, use your testing framework (e.g., Mocha) to define and write unit tests for specific functions or components. Include assertions to check for expected outcomes.
Example (using Mocha and Chai):

Run Unit Tests: Execute your unit tests by running “npm test”. This command runs your test scripts defined in the "scripts" section of your “package.json” file.
Conducting Integration Tests
Create Integration Test Files: In the "tests" directory, create new test files for integration testing specific interactions between modules or components.
Write Integration Tests: Write integration tests in these files to check how different parts of your application work together. Use assertions to verify expected behavior.
Example (using Mocha and Chai):
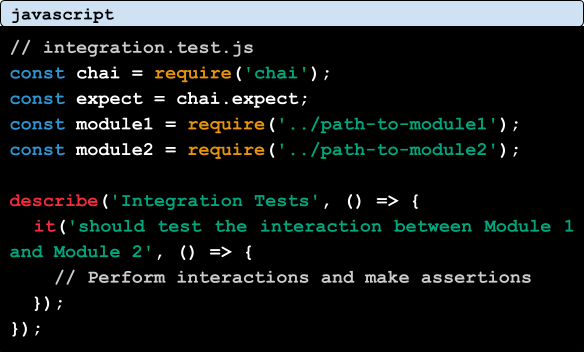
Run Integration Tests: Execute integration tests using the “npm test” command.
Implementing End-to-End Tests
Create End-to-End Test Files: In the "tests" directory, create test files for end-to-end testing. These tests will simulate the entire application's workflow.
Write End-to-End Tests: Write tests that mimic user scenarios, covering complete application workflows from start to finish. Use testing tools like Supertest for HTTP requests to simulate user interactions.
Example (using Supertest and Mocha):

Run End-to-End Tests: Execute end-to-end tests using the “npm test” command.
Best Practices for Node.js Testing
Plan your tests: Outline and plan what to test before writing the code. This can be achieved through test-driven development (TDD) to ensure clear objectives for your code.
Utilize Testing Frameworks: Use testing frameworks like Mocha, Jest, or Jasmine for structuring and organizing your tests, making it easier to run and manage them.
Test Small and Isolated Units: Test individual units or components of your code (like functions or modules) in isolation to ensure they work correctly and independently.
Aim for Good Test Coverage: Strive for high test coverage to ensure that most parts of your code are tested, reducing the risk of undetected issues.
Use Mocks and Stubs: Employ tools like Sinon to create mocks or stubs, allowing you to simulate certain parts of your code without relying on live systems like databases or external services.
Maintain Test Structure: Organize tests into clear sections: arrange, act, and assert (AAA) to set up test conditions, perform actions, and make assertions, making the test structure clear and consistent.
Keep Tests Independent: Ensure each test operates independently, not relying on other tests to pass, to prevent dependencies between tests.
Integrate Tests into CI/CD: Include tests in your continuous integration and deployment process to ensure tests run automatically with each change, catching issues early.
Refactor and Keep Tests Maintainable: Regularly review and refactor tests as your code evolves, ensuring they stay relevant and easy to understand.
Continuous Learning
Stay Updated: Continuously learn about new testing tools, best practices, and advancements in Node.js testing to improve your testing approach.
Engage with the Community: Participate in forums, discussions, and communities to share experiences, learn from others, and stay updated with the latest trends in testing.
Experiment and Adapt: Try new testing approaches, tools, or methodologies to adapt and improve your testing process.
Regular Skill Development: Keep enhancing your testing skills by learning new techniques, tools, and methodologies that can benefit your testing strategy.
Reflect and Improve: Regularly reflect on your testing practices, identify areas for improvement, and adapt your approach to achieve better results.
QAble is a prominent automation testing company in India, offering comprehensive automation testing services. Selecting the perfect automation web testing tool for your product can be tricky. It’s crucial to think about what you need, your budget, and whether it works with your system. We offer a wide range of testing services, including NodeJS testing, to ensure your web apps are top-notch and thoroughly tested for quality.
Discover More About QA Services
sales@qable.ioDelve deeper into the world of quality assurance (QA) services tailored to your industry needs. Have questions? We're here to listen and provide expert insights